MCP TypeScript SDK 1.10.x
- Staff Desk
- 1 day ago
- 5 min read
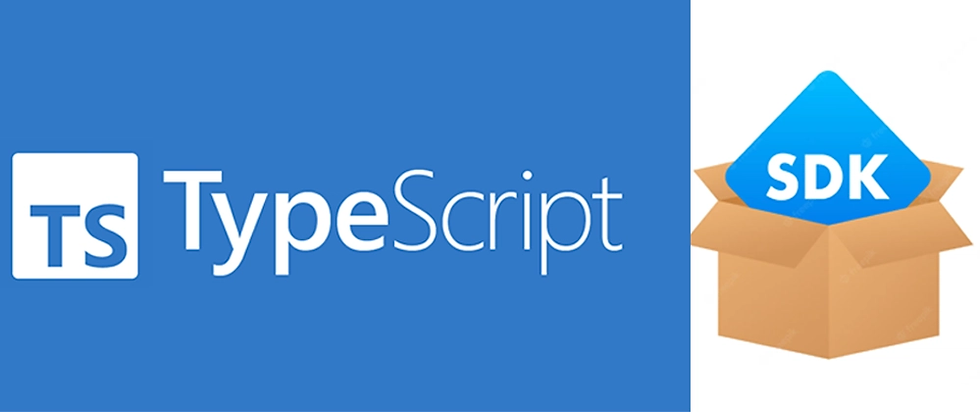
The MCP TypeScript SDK (Software Development Kit) version 1.10.x is a powerful tool for developers looking to integrate Multi-Channel Processing (MCP) functionality into their TypeScript applications. This SDK provides an easy-to-use interface for interacting with MCP servers, enabling seamless integration and powerful data processing capabilities. In this article, we will explore the features, installation, and usage of the MCP TypeScript SDK 1.10.x, as well as its potential applications in building scalable, high-performance applications.
What is MCP TypeScript SDK?
The MCP TypeScript SDK is a library designed to simplify the process of integrating MCP functionality into TypeScript-based applications. MCP, or Multi-Channel Processing, enables the simultaneous execution of tasks across multiple channels, which significantly improves performance and scalability. The SDK provides a set of methods and classes that allow developers to interact with MCP servers and utilize the full potential of parallel processing in their applications.
Key Features of MCP TypeScript SDK 1.10.x
The MCP TypeScript SDK version 1.10.x comes with a number of enhanced features and improvements that make it a versatile tool for developers. Here are some of the key features:
1. Improved Multi-Channel Support
Version 1.10.x introduces enhanced support for multi-channel processing, allowing developers to execute multiple tasks concurrently across various processors or threads. This enables faster data processing and improved system performance, making it ideal for high-throughput applications such as data analytics, machine learning, and real-time systems.
2. Seamless Integration with MCP Servers
The SDK is designed to integrate seamlessly with MCP servers, providing an easy-to-use interface for interacting with these servers. Developers can quickly set up connections, send requests, and retrieve results from the MCP server without the need for complex configuration or manual coding.
3. Real-Time Data Processing
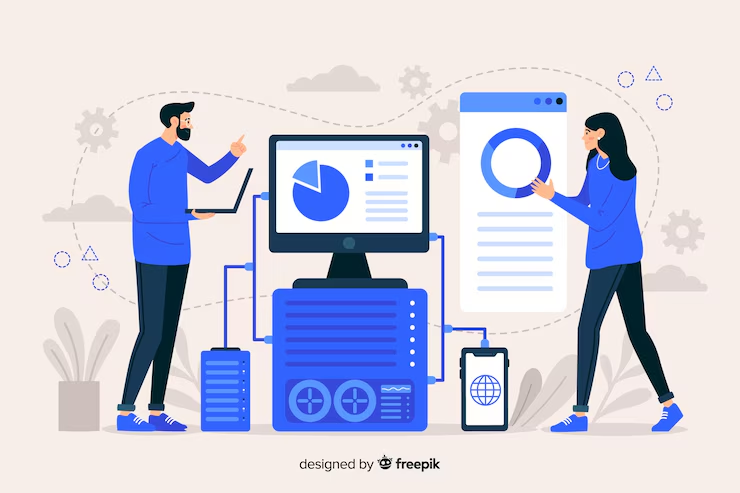
Real-time data processing is a critical feature in many modern applications, and the MCP TypeScript SDK makes it easier than ever to work with real-time data streams. With built-in support for handling large volumes of data in real time, developers can build high-performance applications that can process and analyze data as it comes in.
4. Simplified Task Distribution
The SDK allows developers to easily distribute tasks across multiple processors or systems. By automating task distribution, the SDK reduces the complexity of managing parallel processing and ensures that tasks are completed efficiently, even for large-scale applications.
5. Scalable and Flexible
With built-in scalability features, the MCP TypeScript SDK allows developers to scale their applications as needed. Whether you're building a small application or a large enterprise system, the SDK ensures that your application can handle an increasing number of tasks and workloads without sacrificing performance.
Installing MCP TypeScript SDK 1.10.x
To get started with the MCP TypeScript SDK 1.10.x, you'll need to install it in your project. Here's how you can do that:
1. Install via NPM
The easiest way to install the MCP TypeScript SDK is via NPM (Node Package Manager). Open your terminal or command prompt and run the following command:
npm install mcp-typescript-sdk
This will download and install the SDK along with its dependencies. Once installed, you can import the SDK into your TypeScript project and start using its features.
2. Install via Yarn
If you're using Yarn as your package manager, you can install the MCP TypeScript SDK by running the following command:
yarn add mcp-typescript-sdk
After installation, you can begin utilizing the SDK in your project.
Getting Started with MCP TypeScript SDK
Once you've installed the MCP TypeScript SDK, you're ready to start integrating it into your application. Here's a basic example of how to get started:
Example 1: Connecting to an MCP Server
In this example, we'll show how to establish a connection to an MCP server and send a simple request:
import { MCPClient } from 'mcp-typescript-sdk';
// Create an instance of the MCPClient
const client = new MCPClient({ serverUrl: 'http://localhost:3000' });
// Send a request to the MCP server
client.sendRequest({ task: 'processData', data: { id: 123 } })
.then(response => {
console.log('MCP Server Response:', response);
})
.catch(error => {
console.error('Error:', error);
});
In this example, we create an instance of the MCPClient class, specifying the server URL. We then send a request to the server, passing in some data for processing. The server’s response is logged to the console.
Example 2: Multi-Channel Task Execution
One of the primary features of MCP is the ability to execute tasks across multiple channels simultaneously. Here’s an example that demonstrates how to distribute tasks across channels:
import { MCPClient, Task } from 'mcp-typescript-sdk';
// Create an instance of the MCPClient
const client = new MCPClient({ serverUrl: 'http://localhost:3000' });
// Create tasks to be executed in parallel
const tasks: Task[] = [
{ task: 'processData', data: { id: 123 } },
{ task: 'processData', data: { id: 456 } },
{ task: 'processData', data: { id: 789 } }
];
// Send multiple tasks concurrently
client.sendMultipleRequests(tasks)
.then(responses => {
responses.forEach(response => {
console.log('Task Response:', response);
});
})
.catch(error => {
console.error('Error:', error);
});
In this example, we create multiple tasks and send them concurrently to the MCP server using the `sendMultipleRequests` method. The server processes the tasks in parallel, and the responses are logged to the console.
Advanced Features
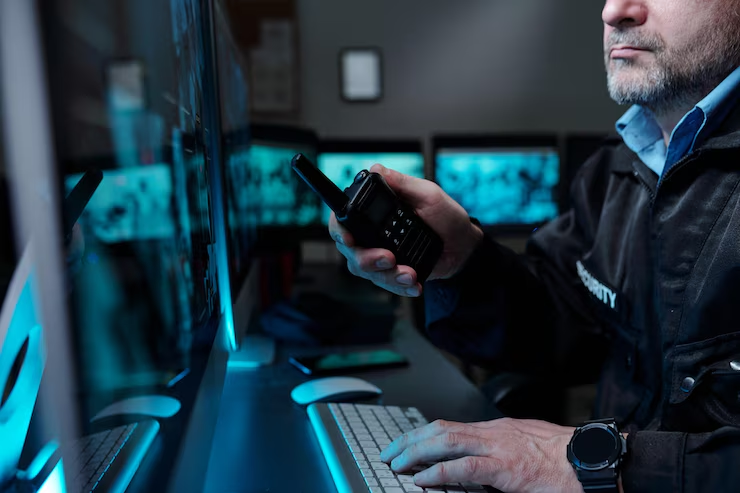
As you become more familiar with the MCP TypeScript SDK, you can explore more advanced features:
1. Error Handling
The SDK provides robust error handling, allowing developers to catch and handle errors effectively. Whether it’s a network issue or a server error, the SDK ensures that your application can recover gracefully.
2. Authentication and Security
For applications that require secure communication with MCP servers, the SDK offers built-in support for authentication and encryption. You can easily set up secure connections and ensure that sensitive data is transmitted safely.
3. Logging and Debugging
The SDK includes logging features that allow you to track requests, responses, and errors. These logs can help you debug your application and monitor its performance in real time.
Use Cases for MCP TypeScript SDK 1.10.x
The MCP TypeScript SDK is versatile and can be used in a variety of applications, including:
Real-Time Data Processing: Process large streams of data across multiple channels in real time, ideal for IoT applications or financial data analysis.
Distributed Computing: Offload computationally intensive tasks to multiple processors or systems, improving efficiency and performance.
Machine Learning: Use the SDK to parallelize training or inference tasks across multiple systems, speeding up machine learning workflows.
API Integration: Integrate the SDK with third-party APIs to distribute tasks and data across multiple services simultaneously.
Conclusion
The MCP TypeScript SDK 1.10.x is a powerful tool for developers who want to integrate Multi-Channel Processing capabilities into their TypeScript applications. With features such as parallel task execution, real-time data processing, and easy integration with MCP servers, the SDK provides everything you need to build high-performance, scalable applications. Whether you’re working on big data processing, machine learning, or distributed systems, the MCP TypeScript SDK offers the tools necessary to unlock the full potential of your application.
Comments